Premium Only Content
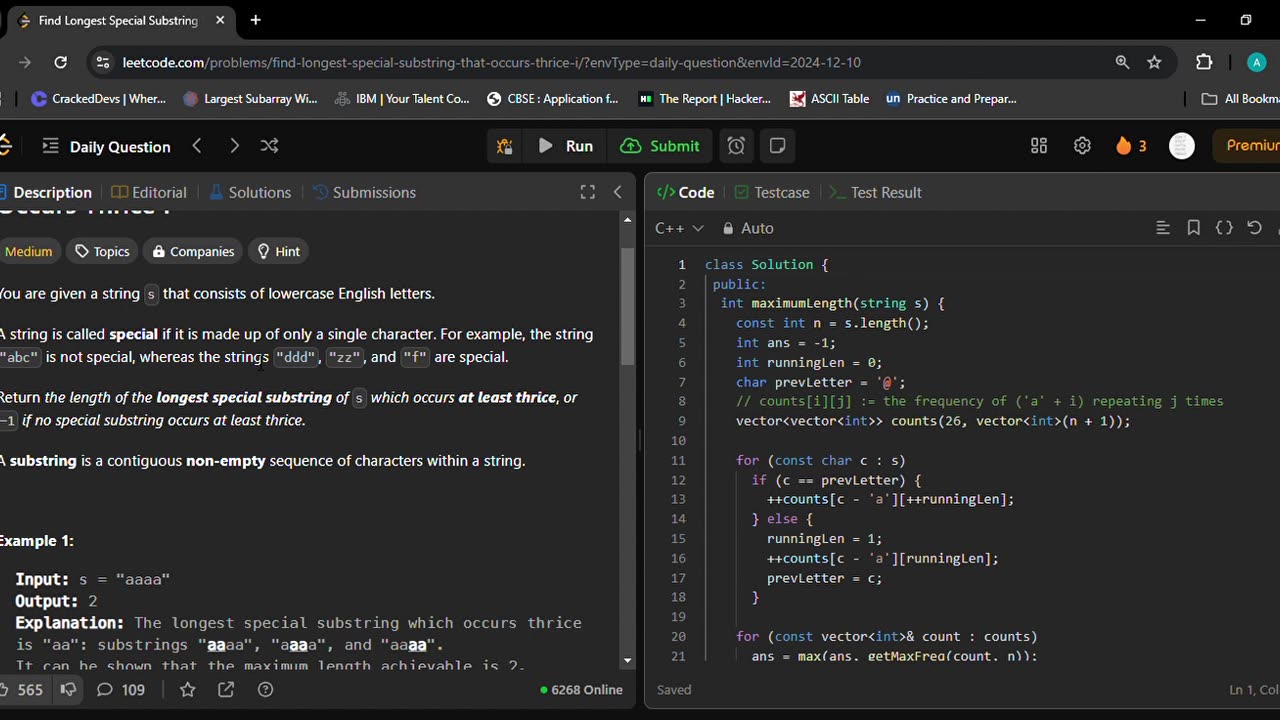
2981. Find Longest Special Substring That Occurs Thrice I
You are given a string s that consists of lowercase English letters.
A string is called special if it is made up of only a single character. For example, the string "abc" is not special, whereas the strings "ddd", "zz", and "f" are special.
Return the length of the longest special substring of s which occurs at least thrice, or -1 if no special substring occurs at least thrice.
A substring is a contiguous non-empty sequence of characters within a string.
Example 1:
Input: s = "aaaa"
Output: 2
Explanation: The longest special substring which occurs thrice is "aa": substrings "aaaa", "aaaa", and "aaaa".
It can be shown that the maximum length achievable is 2.
Example 2:
Input: s = "abcdef"
Output: -1
Explanation: There exists no special substring which occurs at least thrice. Hence return -1.
Example 3:
Input: s = "abcaba"
Output: 1
Explanation: The longest special substring which occurs thrice is "a": substrings "abcaba", "abcaba", and "abcaba".
It can be shown that the maximum length achievable is 1.
Constraints:
3 <= s.length <= 50
s consists of only lowercase English letters.
class Solution {
public:
int maximumLength(string s) {
const int n = s.length();
int ans = -1;
int runningLen = 0;
char prevLetter = '@';
// counts[i][j] := the frequency of ('a' + i) repeating j times
vector<vector<int>> counts(26, vector<int>(n + 1));
for (const char c : s)
if (c == prevLetter) {
++counts[c - 'a'][++runningLen];
} else {
runningLen = 1;
++counts[c - 'a'][runningLen];
prevLetter = c;
}
for (const vector<int>& count : counts)
ans = max(ans, getMaxFreq(count, n));
return ans;
}
private:
// Returns the maximum frequency that occurs more than three times.
int getMaxFreq(const vector<int>& count, int maxFreq) {
int times = 0;
for (int freq = maxFreq; freq >= 1; --freq) {
times += count[freq];
if (times >= 3)
return freq;
}
return -1;
}
};
-
LIVE
vivafrei
50 minutes agoGavin Newsom's War With Elon! Stolen Humvees FOUND? Arson Suspect IDENTIFIED? Cali Updates & MORE!
3,500 watching -
LIVE
Nerdrotic
1 hour agoReconstructing the Superhero | FNT Square Up - Nerdrotic Nooner 457
1,141 watching -
LIVE
The Charlie Kirk Show
5 minutes agoCA's Top Priority + Seven Days | Sen. Marshall, George, Thibeau | 1.13.2025
1,561 watching -
1:04:08
Russell Brand
2 hours agoDeep State Exposed: Veterans in the Crosshairs – SF518
33.7K33 -
LIVE
Game On!
1 hour agoNFL Wildcard Weekend FINALE! Plus, can Notre Dame actually beat Ohio State?
97 watching -
1:00:13
The Dan Bongino Show
5 hours agoThe FBI Warns Of A Grave Threat, But Will Anyone Believe Them? (Ep. 2399) - 01/13/2025
536K929 -
1:23:10
The Rubin Report
2 hours agoMark Zuckerberg Makes Joe Rogan Go Quiet with Never-Before-Told Details of Biden’s Lies
35.1K36 -
LIVE
Grant Stinchfield
1 hour agoBe Wary of Viral CA Wildfire Conspiracies... But the Anomalies Can't be Ignored!
329 watching -
LIVE
The Dana Show with Dana Loesch
1 hour agoThe Dana Show | 1-13-25
595 watching -
LIVE
The Shannon Joy Show
4 hours ago🔥🔥The LA Fires & OMNI War. The Enemies Are Within & The Rules Have Changed.🔥🔥
409 watching