Premium Only Content
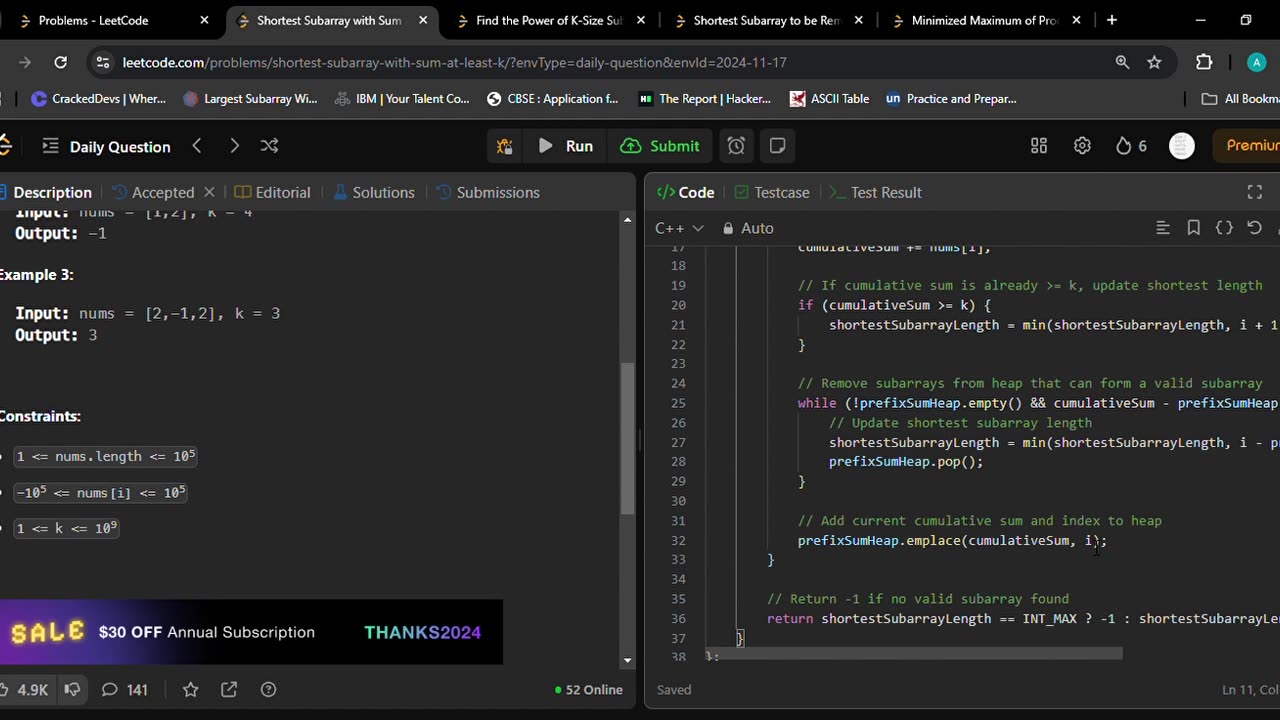
862. Shortest Subarray with Sum at Least K
Given an integer array nums and an integer k, return the length of the shortest non-empty subarray of nums with a sum of at least k. If there is no such subarray, return -1.
A subarray is a contiguous part of an array.
Example 1:
Input: nums = [1], k = 1
Output: 1
Example 2:
Input: nums = [1,2], k = 4
Output: -1
Example 3:
Input: nums = [2,-1,2], k = 3
Output: 3
Constraints:
1 <= nums.length <= 105
-105 <= nums[i] <= 105
1 <= k <= 109
class Solution {
public:
int shortestSubarray(vector<int>& nums, int k) {
int n = nums.size();
// Initialize result to the maximum possible integer value
int shortestSubarrayLength = INT_MAX;
long long cumulativeSum = 0;
// Min-heap to store cumulative sum and its corresponding index
priority_queue<pair<long long, int>, vector<pair<long long, int>>,greater<>> prefixSumHeap;
// Iterate through the array
for (int i = 0; i < n; i++) {
// Update cumulative sum
cumulativeSum += nums[i];
// If cumulative sum is already >= k, update shortest length
if (cumulativeSum >= k) {
shortestSubarrayLength = min(shortestSubarrayLength, i + 1);
}
// Remove subarrays from heap that can form a valid subarray
while (!prefixSumHeap.empty() && cumulativeSum - prefixSumHeap.top().first >= k) {
// Update shortest subarray length
shortestSubarrayLength = min(shortestSubarrayLength, i - prefixSumHeap.top().second);
prefixSumHeap.pop();
}
// Add current cumulative sum and index to heap
prefixSumHeap.emplace(cumulativeSum, i);
}
// Return -1 if no valid subarray found
return shortestSubarrayLength == INT_MAX ? -1 : shortestSubarrayLength;
}
};
-
LIVE
FreshandFit
2 hours agoJoe Budden Arrested For Being A Perv! Tesla Cybertruck Explosion
4,961 watching -
DVR
Kim Iversen
5 hours agoNew Year, New PSYOP?: The Fort Bragg Connection In The New Years Terror Attacks
14.2K64 -
1:41:18
Glenn Greenwald
4 hours agoTerror Attacks Exploited To Push Unrelated Narratives; Facing Imminent Firing Squad, Liz Cheney Awarded Presidential Medal | SYSTEM UPDATE #381
51K63 -
LIVE
Man in America
6 hours ago🔴 LIVE: Terror Attacks or False Flags? IT DOESN'T ADD UP!!!
1,111 watching -
1:02:38
Donald Trump Jr.
8 hours agoNew Year’s Terror, Latest Breaking News with Sebastian Gorka | TRIGGERED Ep.204
128K270 -
59:59
The StoneZONE with Roger Stone
3 hours agoAfter Years of Targeting Trump, FBI and DOJ are Unprepared to Stop Terror Attacks | The StoneZONE
19.2K5 -
1:26:42
Leonardaisfunny
1 hour ago $0.79 earnedH-1b Visas: Infinity Indians
5.06K17 -
1:08:33
Josh Pate's College Football Show
6 hours ago $0.33 earnedPlayoff Reaction Special: Ohio State Owns Oregon | Texas Survives | UGA vs Notre Dame Takeaways
4.9K1 -
58:04
Kimberly Guilfoyle
6 hours agoFBI's Terror Response Failures, Live with Steve Friend & Kyle Seraphin | Ep. 185
84.8K36 -
2:15:01
WeAreChange
7 hours agoMassive Developments In Vegas Investigation! UNREAL DETONATION, Shocking Details Emerge!
92.2K27