Premium Only Content
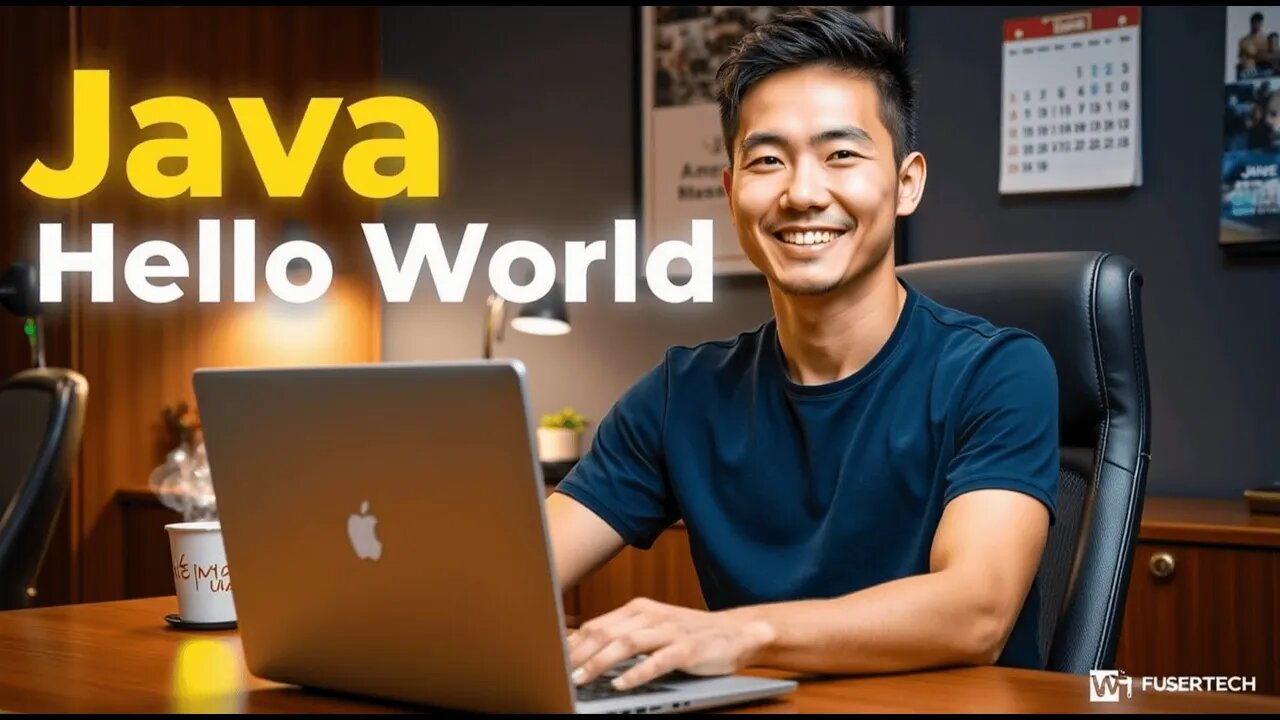
Java "Hello World"
Java Tutorial 1: Printing "Hello, World!"
This tutorial covers the very first step in Java programming: printing "Hello, World!" on the screen. This simple program introduces the structure of a Java program and demonstrates how to display text in the console.
Writing the Code:
In your HelloWorld.java file, type the following code:
java
Copy code
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Understanding the Code:
public class HelloWorld: Defines a class named HelloWorld. In Java, every program must have at least one class, and the file name must match the class name.
public static void main(String[] args): This is the main method, the entry point of every Java application. When you run the program, this method is executed.
System.out.println("Hello, World!");: This line prints "Hello, World!" to the console. The System.out.println method outputs text to the screen, followed by a new line.
Compiling and Running the Program:
Compile the code by opening a terminal, navigating to the folder where HelloWorld.java is saved, and running:
bash
Copy code
javac HelloWorld.java
This command compiles the code and creates a file named HelloWorld.class.
Run the program with:
bash
Copy code
java HelloWorld
You should see the output:
Copy code
Hello, World!Writing the Code:
In your HelloWorld.java file, type the following code:
java
Copy code
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Understanding the Code:
public class HelloWorld: Defines a class named HelloWorld. In Java, every program must have at least one class, and the file name must match the class name.
public static void main(String[] args): This is the main method, the entry point of every Java application. When you run the program, this method is executed.
System.out.println("Hello, World!");: This line prints "Hello, World!" to the console. The System.out.println method outputs text to the screen, followed by a new line.
Compiling and Running the Program:
Compile the code by opening a terminal, navigating to the folder where HelloWorld.java is saved, and running:
bash
Copy code
javac HelloWorld.java
This command compiles the code and creates a file named HelloWorld.class.
Run the program with:
bash
Copy code
java HelloWorld
You should see the output:
Copy code
Hello, World!
-
34:38
Tudor Dixon
3 hours agoThe Changing Landscape Between Tech and Politics with Mike Benz | The Tudor Dixon Podcast
19.6K1 -
2:23:58
Matt Kohrs
14 hours agoRumble's Stock Is EXPLODING!!! || The MK Show
68.6K6 -
1:57:47
LFA TV
17 hours agoBOMBSHELL FINAL REPORT: BIDEN ADMIN SUPPRESSED WUHAN LAB LEAK | LIVE FROM AMERICA 12.27.24 11am EST
43.5K6 -
43:07
Grant Stinchfield
3 hours ago $4.64 earnedWe Built it... China Controls it... Trump Will Take it Back!
21.3K7 -
35:05
Rethinking the Dollar
3 hours agoTime to Pay the Piper! Debt Refinance Crisis Coming in 2025
20.4K3 -
52:34
PMG
17 hours ago $4.23 earnedHannah Faulkner and Steve Friend | EXPOSE THE FBI CORRUPTION - KASH PATEL
16.1K8 -
2:58:58
Wendy Bell Radio
9 hours agoOn Day One
89.8K78 -
1:59:27
Jeff Ahern
6 hours ago $3.99 earnedFriday Freak out with Jeff Ahern (6am Pacific)
31.2K1 -
1:56:07
Game On!
14 hours ago $1.72 earnedJosh Allen is the NFL MVP! It's not even close!
24.5K2 -
13:05
Neil McCoy-Ward
5 hours agoWhy The Media Won't Tell You What Milei Did In Argentina...
24.3K1