Premium Only Content
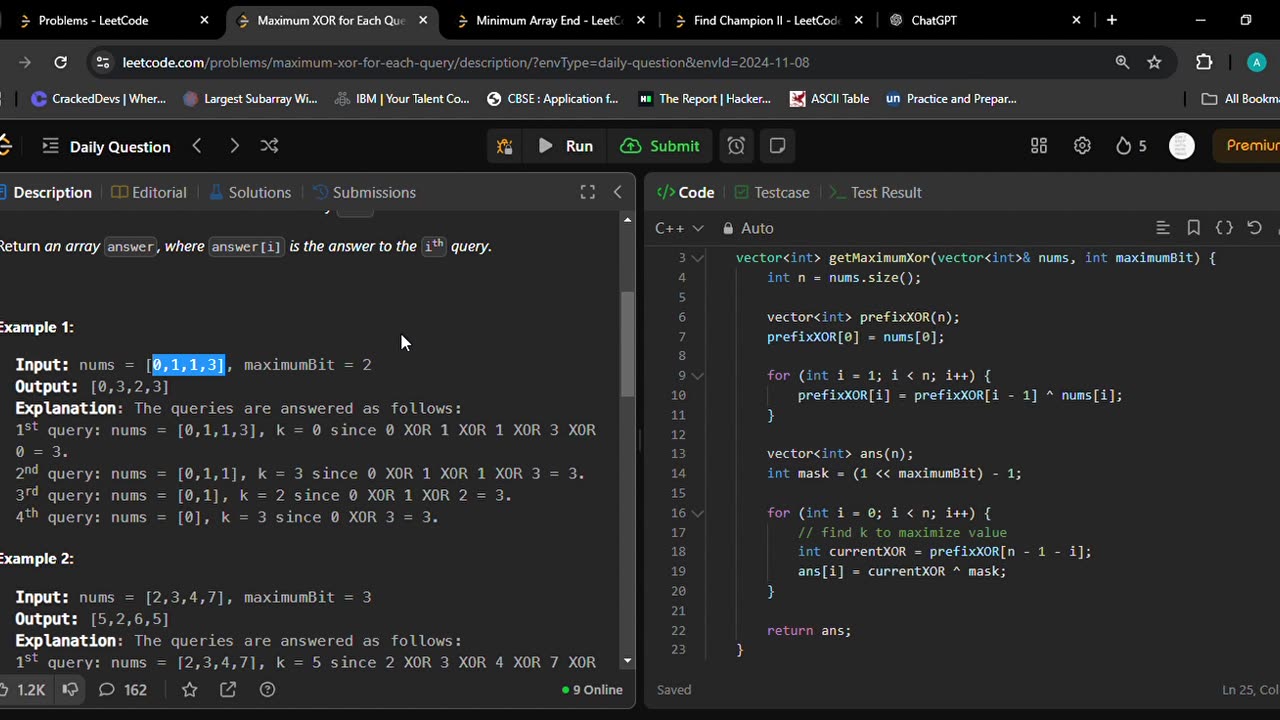
1829. Maximum XOR for Each Query
You are given a sorted array nums of n non-negative integers and an integer maximumBit. You want to perform the following query n times:
Find a non-negative integer k < 2maximumBit such that nums[0] XOR nums[1] XOR ... XOR nums[nums.length-1] XOR k is maximized. k is the answer to the ith query.
Remove the last element from the current array nums.
Return an array answer, where answer[i] is the answer to the ith query.
Example 1:
Input: nums = [0,1,1,3], maximumBit = 2
Output: [0,3,2,3]
Explanation: The queries are answered as follows:
1st query: nums = [0,1,1,3], k = 0 since 0 XOR 1 XOR 1 XOR 3 XOR 0 = 3.
2nd query: nums = [0,1,1], k = 3 since 0 XOR 1 XOR 1 XOR 3 = 3.
3rd query: nums = [0,1], k = 2 since 0 XOR 1 XOR 2 = 3.
4th query: nums = [0], k = 3 since 0 XOR 3 = 3.
Example 2:
Input: nums = [2,3,4,7], maximumBit = 3
Output: [5,2,6,5]
Explanation: The queries are answered as follows:
1st query: nums = [2,3,4,7], k = 5 since 2 XOR 3 XOR 4 XOR 7 XOR 5 = 7.
2nd query: nums = [2,3,4], k = 2 since 2 XOR 3 XOR 4 XOR 2 = 7.
3rd query: nums = [2,3], k = 6 since 2 XOR 3 XOR 6 = 7.
4th query: nums = [2], k = 5 since 2 XOR 5 = 7.
Example 3:
Input: nums = [0,1,2,2,5,7], maximumBit = 3
Output: [4,3,6,4,6,7]
Constraints:
nums.length == n
1 <= n <= 105
1 <= maximumBit <= 20
0 <= nums[i] < 2maximumBit
nums is sorted in ascending order.
class Solution {
public:
vector<int> getMaximumXor(vector<int>& nums, int maximumBit) {
int n = nums.size();
vector<int> prefixXOR(n);
prefixXOR[0] = nums[0];
for (int i = 1; i < n; i++) {
prefixXOR[i] = prefixXOR[i - 1] ^ nums[i];
}
vector<int> ans(n);
int mask = (1 << maximumBit) - 1;
for (int i = 0; i < n; i++) {
// find k to maximize value
int currentXOR = prefixXOR[n - 1 - i];
ans[i] = currentXOR ^ mask;
}
return ans;
}
};
-
DVR
Kim Iversen
5 hours agoNew Year, New PSYOP?: The Fort Bragg Connection In The New Years Terror Attacks
14.2K64 -
1:41:18
Glenn Greenwald
4 hours agoTerror Attacks Exploited To Push Unrelated Narratives; Facing Imminent Firing Squad, Liz Cheney Awarded Presidential Medal | SYSTEM UPDATE #381
51K59 -
LIVE
Man in America
6 hours ago🔴 LIVE: Terror Attacks or False Flags? IT DOESN'T ADD UP!!!
1,042 watching -
1:02:38
Donald Trump Jr.
8 hours agoNew Year’s Terror, Latest Breaking News with Sebastian Gorka | TRIGGERED Ep.204
128K257 -
59:59
The StoneZONE with Roger Stone
3 hours agoAfter Years of Targeting Trump, FBI and DOJ are Unprepared to Stop Terror Attacks | The StoneZONE
19.2K5 -
1:26:42
Leonardaisfunny
1 hour ago $0.79 earnedH-1b Visas: Infinity Indians
5.06K17 -
1:08:33
Josh Pate's College Football Show
6 hours ago $0.33 earnedPlayoff Reaction Special: Ohio State Owns Oregon | Texas Survives | UGA vs Notre Dame Takeaways
4.9K1 -
58:04
Kimberly Guilfoyle
6 hours agoFBI's Terror Response Failures, Live with Steve Friend & Kyle Seraphin | Ep. 185
84.8K36 -
2:15:01
WeAreChange
7 hours agoMassive Developments In Vegas Investigation! UNREAL DETONATION, Shocking Details Emerge!
92.2K27 -
54:02
LFA TV
13 hours ago2025 Is Off to a Violent Start | TRUMPET DAILY 1.2.25 7pm
32.5K5