Premium Only Content
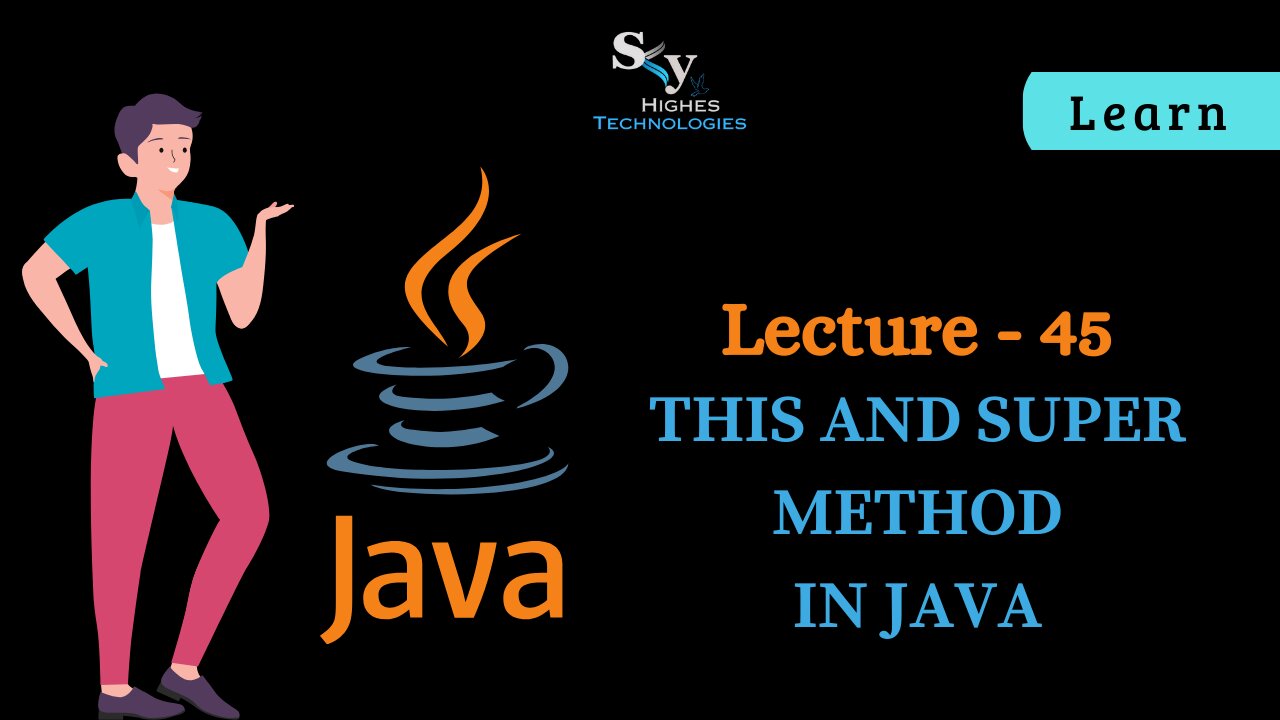
#45 This and Super Method in Java | Skyhighes | Lecture 45
Here's a comprehensive explanation of the this and super keywords in Java, focusing on their use within methods:
this Keyword
Within a method, this refers to the current object—the object whose method is being called. It has several uses:
Referencing instance variables: To distinguish between local variables and instance variables with the same name:
Java
public class Person {
private String name;
public void setName(String name) {
this.name = name; // Refers to the instance variable
}
}
Use code with caution. Learn more
Calling other methods of the same object: To invoke another method of the current object:
Java
public class Person {
public void introduce() {
System.out.println("Hello, my name is " + this.getName());
}
}
Use code with caution. Learn more
Passing the current object as an argument: To pass the current object as a reference to another method:
Java
public class Person {
public void register(Event event) {
event.addParticipant(this); // Passing the current Person object
}
}
Use code with caution. Learn more
super Keyword
Within a method, super refers to the immediate parent class of the current object. It's primarily used to:
Access superclass members: To access members (variables or methods) of the superclass that have been hidden or overridden:
Java
public class Employee extends Person {
public String getDetails() {
return super.getName() + ", works in IT"; // Accessing superclass method
}
}
Use code with caution. Learn more
Call superclass constructors: To explicitly call a constructor of the superclass from a subclass constructor:
Java
public class Employee extends Person {
public Employee(String name, String jobTitle) {
super(name); // Calling superclass constructor
this.jobTitle = jobTitle;
}
}
Use code with caution. Learn more
Key Points:
this and super are both reserved keywords in Java.
They are used within methods to refer to different objects within the inheritance hierarchy.
this is used for accessing or calling members of the current object.
super is used for accessing or calling members of the superclass.
Understanding these keywords is essential for working with inheritance and object relationships in Java.
-
LIVE
WeAreChange
2 hours agoElon Musk & Donald Trump: The Emergency Halt That Saved Us
2,924 watching -
LIVE
LFA TV
22 hours agoThe German Strongman’s Arrival Is Imminent | Trumpet Daily 12.18.24 7PM EST
549 watching -
LIVE
Melonie Mac
2 hours agoGo Boom Live Ep 32! Soul Reaver Remastered!
357 watching -
LIVE
Sarah Westall
36 minutes agoDigital Slavery and Playing with Fire: Money, Banking, and the Federal Reserve w/ Tom DiLorenzo
439 watching -
LIVE
2 MIKES LIVE
5 hours ago2 MIKES LIVE #157 ILLEGALS, PROTESTORS AND DRONES!
147 watching -
1:01:03
LFA TV
22 hours agoTHE LATEST SPENDING BILL IS AN ABOMINATION! | UNGOVERNED 12.18.24 5pm EST
7.51K8 -
1:43:34
Redacted News
4 hours agoBREAKING! WARMONGERS PUSHING TRUMP TO LAUNCH PRE-EMPTIVE WAR WITH IRAN | Redacted News
82.8K182 -
1:00:26
Candace Show Podcast
3 hours agoPiers Morgan x Candace Owens | Candace Ep 123
40.9K142 -
2:06:51
Darkhorse Podcast
6 hours agoThe 256th Evolutionary Lens with Bret Weinstein and Heather Heying
41.2K24 -
3:08:08
Scammer Payback
4 hours agoCalling Scammer Live
22K1