Premium Only Content
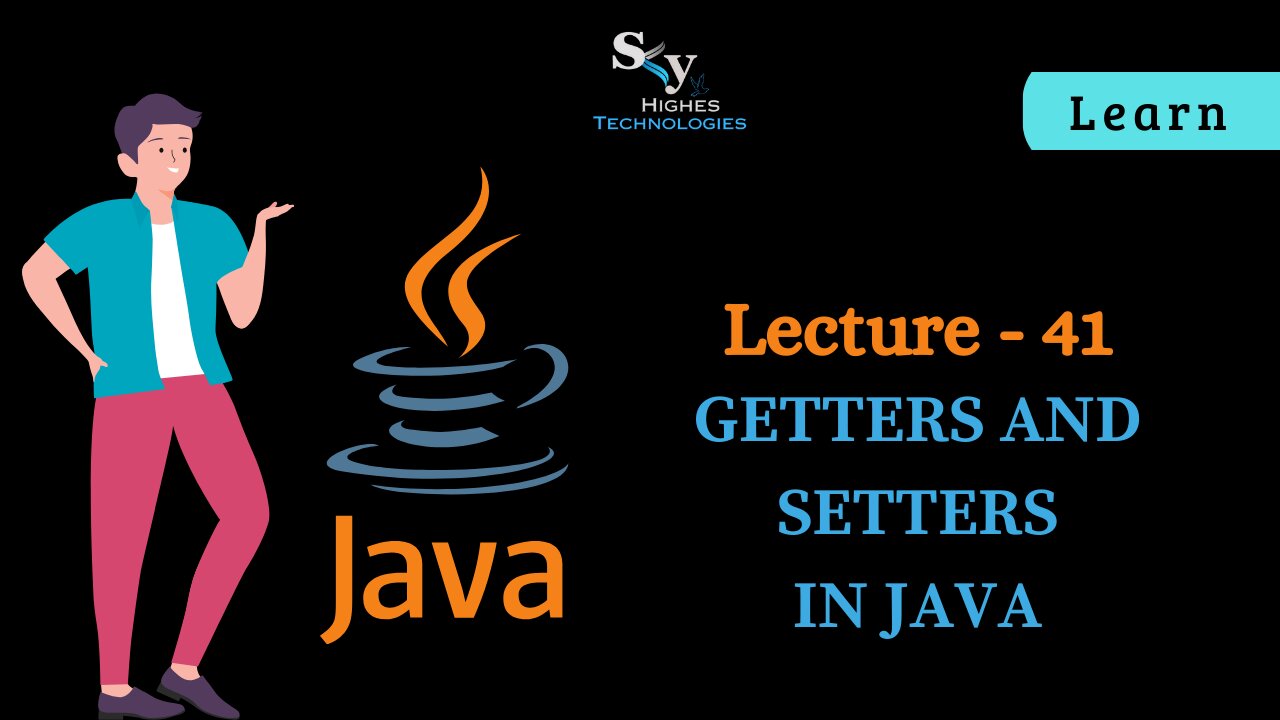
#41 Getter and Setter in JAVA | Syhighes | Lecture 41
Getters and Setters in Java
Getters and setters are fundamental building blocks of object-oriented programming in Java. They work together to implement encapsulation, a key concept that protects the internal state of an object and ensures data integrity.
What are Getters?
Getters are public methods that allow controlled access to an object's private data members.
They essentially "get" the value of a private variable and return it to the caller.
Getters typically follow a naming convention like get<VariableName>, for example, getName(), getAge(), or isEligible().
Benefits of Getters:
Data security: Prevents direct access to sensitive data, promoting controlled manipulation.
Encapsulation: Supports data hiding, ensuring the object's internal state remains protected.
Code clarity: Makes code more readable and understandable by providing clear access points to data.
Flexibility: Allows for future modifications to the internal data representation without affecting existing code that uses getters.
What are Setters?
Setters are public methods that allow controlled modification of an object's private data members.
They take a parameter of the same type as the private variable and "set" the value within the object.
Setters typically follow a naming convention like set<VariableName>, for example, setName(String name), setAge(int age), or setIsEligible(boolean eligible).
Benefits of Setters:
Data validation: Enables implementing logic to validate the input value before setting it, ensuring data integrity.
Controlled updates: Prevents unauthorized or accidental modification of sensitive data.
Encapsulation: Supports data hiding by providing a controlled way to update the object's internal state.
Flexibility: Allows for future modifications to the data update logic without affecting existing code that uses setters.
Example:
Java
class
Person
{
private String name;
private
int age;
public String getName()
{
return
name;
}
public
void
setName(String newName)
{
this.name = newName;
}
public
int
getAge()
{
return age;
}
public
void
setAge(int newAge)
{
if (newAge < 0) {
throw new IllegalArgumentException("Age cannot be negative.");
}
this.age = newAge;
}
}
Use code with caution. Learn more
In this example, the Person class has private variables for name and age. The getName and getAge methods are getters that return the values of these variables. The setName and setAge methods are setters that allow modifying the values with validation logic for age.
Using Getters and Setters:
Getters are typically used when you need to read the current value of a data member from outside the object.
Setters are typically used when you need to update the value of a data member from outside the object.
Best Practices:
Use getters and setters for all private data members.
Follow consistent naming conventions.
Implement data validation in setters where necessary.
Avoid unnecessary complexity in getters and setters.
By understanding and using getters and setters effectively, you can write clean, maintainable, and secure object-oriented code in Java.
I hope this explanation with visuals helps! Feel free to ask if you have any further questions.
-
25:59
TampaAerialMedia
1 day ago $1.19 earnedUpdate ANNA MARIA ISLAND 2025
22.3K3 -
59:31
Squaring The Circle, A Randall Carlson Podcast
11 hours ago#039: How Politics & War, Art & Science Shape Our World; A Cultural Commentary From Randall Carlson
17.4K2 -
13:21
Misha Petrov
11 hours agoThe CRINGIEST Thing I Have Ever Seen…
14.5K37 -
11:45
BIG NEM
7 hours agoWe Blind Taste Tested the Best Jollof in Toronto 🇳🇬🇬🇭
9.1K -
15:40
Fit'n Fire
10 hours ago $0.20 earnedArsenal SLR106f & LiteRaider AK Handguard from 1791 Industries
7.82K1 -
8:34
Mike Rowe
6 days agoWhat You Didn't Hear At Pete's Confirmation Hearing | The Way I Heard It with Mike Rowe
45.8K19 -
7:13:44
TonYGaMinG
12 hours ago🟢LATEST! KINGDOM COME DELIVERANCE 2 / NEW EMOTES / BLERPS #RumbleGaming
67.8K4 -
40:17
SLS - Street League Skateboarding
4 days agoEVERY 9 CLUB IN FLORIDA! Looking back at SLS Jacksonville 2021 & 2022 - Yuto, Jagger, Sora & more...
111K1 -
2:00:47
PaddysParlorGames
21 hours agoSunday Parlor Chill: GOBSTEIN
70.1K5 -
LIVE
Major League Fishing
5 days agoLIVE! - Bass Pro Tour: Stage 2 - Day 4
122 watching