Premium Only Content
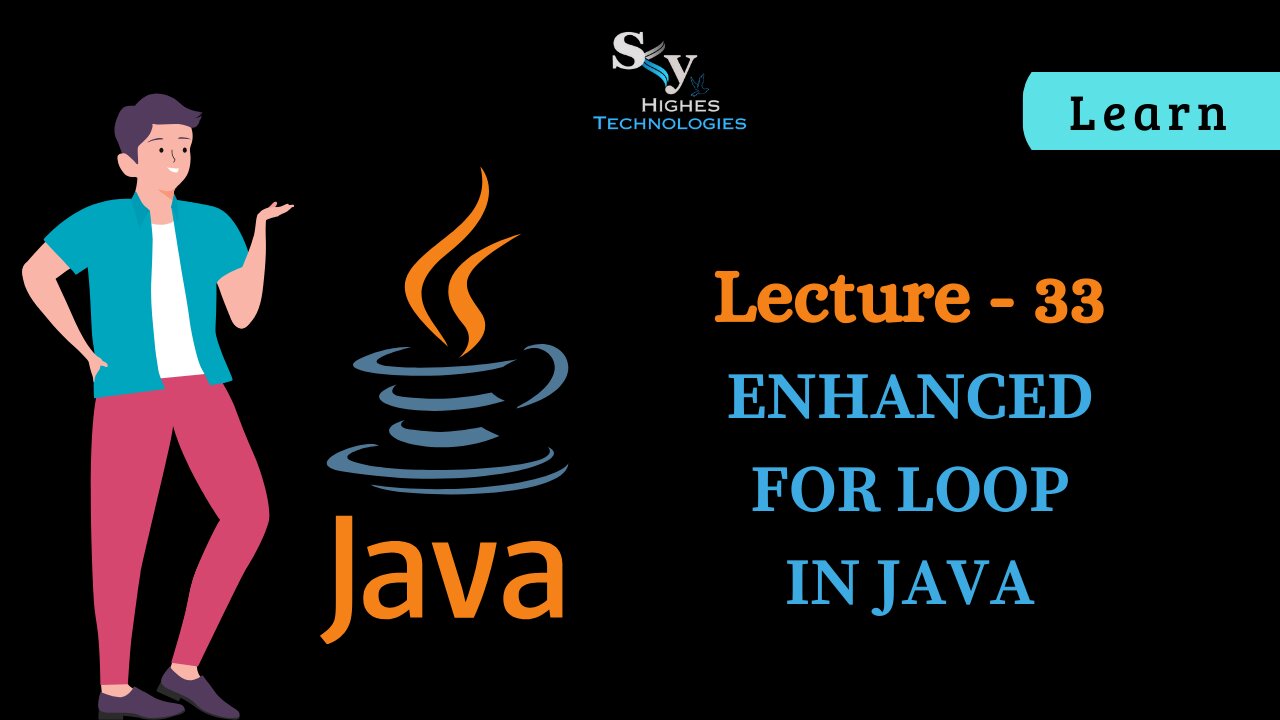
#33 Enhance for Loop in JAVA | Skyhighes | Lecture 33
Here's a breakdown of the enhanced for loop (also known as the for-each loop) in Java:
Purpose:
Simplifies iterating over arrays and collections.
Focuses on the elements themselves rather than index management.
Offers a more concise and readable way to process elements.
Syntax:
Java
for (elementType variableName : arrayOrCollection) {
// code to execute for each element
}
Use code with caution. Learn more
Breakdown:
elementType: The data type of the elements in the array or collection.
variableName: A variable to hold each element during iteration.
arrayOrCollection: The array or collection you want to iterate over.
Example:
Java
int[] numbers = {10, 20, 30, 40};
for (int num : numbers) {
System.out.println(num); // Prints each number on a separate line
}
Use code with caution. Learn more
Key Points:
No index management: You don't need to track or manage index variables.
Read-only access: The loop variable is read-only, preventing accidental modifications.
Suitable for simple iterations: Ideal for accessing and processing each element without altering the array structure.
Not for index-based operations: If you need to modify elements based on their indices or perform operations that require indices, use a traditional for loop.
Benefits:
Conciseness and readability: Cleaner syntax, making code easier to understand.
Reduced error-proneness: Fewer chances for off-by-one errors or index-related issues.
Focus on elements: Emphasizes the data rather than the underlying structure.
Common Use Cases:
Printing or displaying elements.
Performing simple calculations or transformations on elements.
Searching for specific elements.
Copying elements to another collection.
Remember:
Enhanced for loops are a powerful tool for efficient and readable iteration, but choose the appropriate loop type based on your specific needs.
-
1:01:26
Glenn Greenwald
4 hours agoRapid Fire: Canada Elections, Dem's Sit-In, Israeli Taking Points Escalate; PLUS: Jewish Academics Push-Back on Antisemitism Claims | SYSTEM UPDATE #445
84.6K81 -
DVR
RiftTV/Slightly Offensive
3 hours ago $4.20 earnedTotal Indian TAKEOVER: Has The WEST Completely FALLEN? | Guest: Josh Denny
18.1K10 -
9:46
Mrgunsngear
2 hours ago $4.66 earnedHow To Turn Your Glock Into A PCC
23.4K4 -
16:12
T-SPLY
11 hours agoJeff Bezos Is Now Enemy #1 For The Trump Administration
86.1K68 -
12:24
Tundra Tactical
3 hours ago $1.38 earnedThe SIG Roast to ND Them All
33.1K7 -
1:02:31
BonginoReport
6 hours agoDeportations Keep “Frightened” Michelle Obama Awake at Night (Ep. 37) - Nightly Scroll with Hayley
115K160 -
LIVE
Adam Does Movies
2 days ago $1.24 earnedTalking Movie News & Just Chatting About Films - LIVE!
243 watching -
LIVE
Anthony Rogers
1 day agoEpisode 364 - JFK FILES
63 watching -
1:41:02
megimu32
3 hours agoON THE SUBJECT: 1 Million Views Party! Diddy Drama, Marvel Weirdness, and Total Prom Chaos
19K7 -
1:18:44
Kim Iversen
6 hours agoMagnetic Pole Shift: Europe’s Blackout Is Just the Beginning | 90° Earth Flip Coming
102K222