Premium Only Content
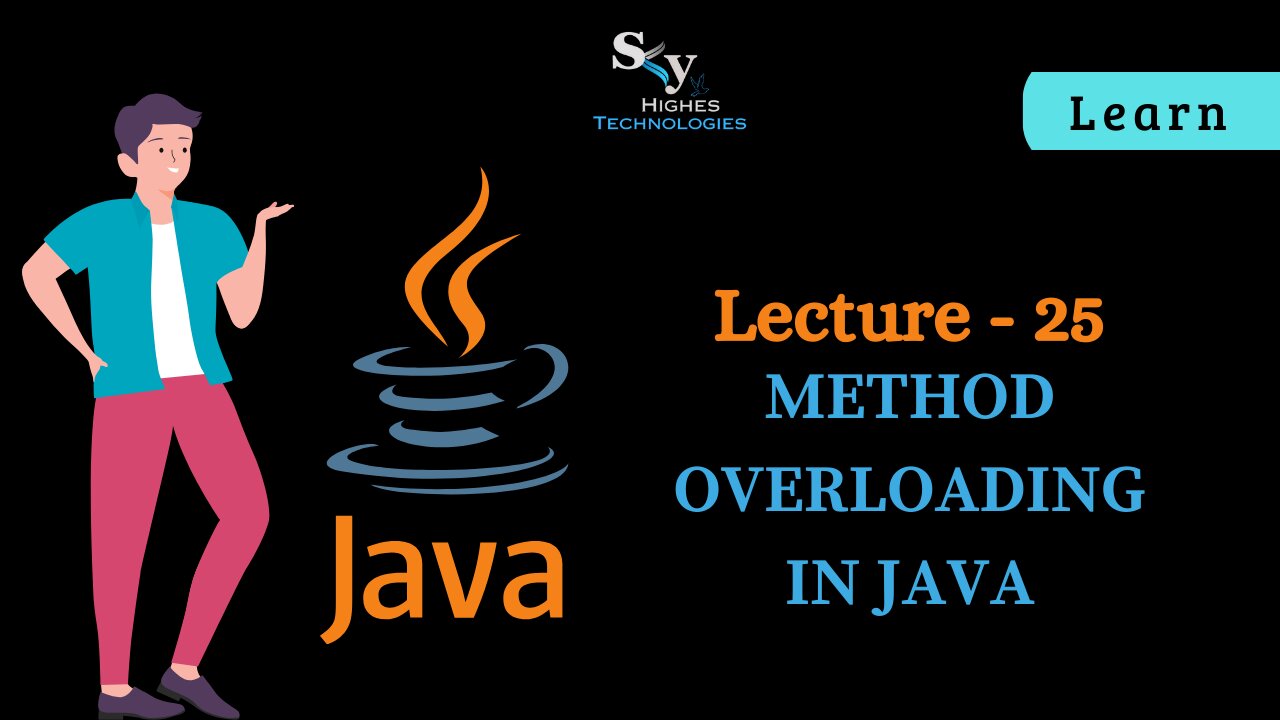
#25 Methods Overloading in JAVA | Skyhighes | Lecture 25
Here's a comprehensive explanation of method overloading in Java, incorporating visuals:
Method Overloading: Same Name, Different Tasks
In Java, method overloading allows you to have multiple methods with the same name within a class, as long as their parameter lists are different. This means they can accept different types or numbers of arguments, enabling flexible code that handles varied inputs.
Key Concepts:
Parameter List Difference: Overloaded methods must have distinct parameter lists, differing in:
Number of parameters
Data types of parameters
Order of parameters
Return Type Irrelevance: Return types alone don't differentiate overloaded methods.
Access Modifiers: Overloaded methods can have different access modifiers (public, private, protected).
Example:
Java
public
class
Calculator
{
public
int
add(int a, int b)
{
return a + b;
}
public
double
add(double a, double b)
{
return a + b;
}
public
int
add(int a, int b, int c)
{
return a + b + c;
}
}
Use code with caution. Learn more
Visualizing Method Overloading:
Benefits of Method Overloading:
Improved Readability: Code becomes more intuitive as methods share a logical name for similar actions.
Type Flexibility: Handles various data types seamlessly.
Code Reusability: Avoids redundant method names for similar operations.
Example Usage:
Java
Calculator calc = new Calculator();
int sum1 = calc.add(5, 3); // Calls int add(int, int)
double sum2 = calc.add(2.5, 1.8); // Calls double add(double, double)
int sum3 = calc.add(1, 2, 3); // Calls int add(int, int, int)
-
1:58:03
Tucker Carlson
3 hours agoJeffrey Sachs: The Inevitable War With Iran, and Biden’s Attempts to Sabotage Trump
103K166 -
1:31:00
Redacted News
6 hours agoBREAKING! Trump demands answers on UFOs over America as Pentagon hides the truth | Redacted News
145K229 -
1:07:45
BIG NEM
4 hours agoSpiritual STDs, Nikola Tesla & Harnessing Creative Energy! ⚡💡
4.76K -
38:09
Patriots With Grit
3 hours agoWe Must Finish This Fight | Glenn Baker
3.84K -
54:52
LFA TV
1 day agoWhy Did God Bring Donald Trump Back to the White House? | Trumpet Daily 12.16.24 7PM EST
21.1K4 -
1:33:00
2 MIKES LIVE
9 hours ago2 MIKES LIVE #156 Author Frank Lasee will Deep Dive into CLIMATE CHANGE!
11.7K1 -
15:36
DeVory Darkins
1 day ago $29.74 earnedGLOVES OFF: Scott Jennings hits CNN Panel with knockout blow
79.4K84 -
28:05
Scammer Payback
11 hours agoHacking the World's Largest Streamer
36.4K5 -
2:16:05
Barry Cunningham
8 hours agoTRUMP DAILY BRIEFING: Can The Trump Train Be Stopped? So Much Winning!
42.1K22 -
1:03:09
In The Litter Box w/ Jewels & Catturd
1 day agoPardoner-in-Chief | In the Litter Box w/ Jewels & Catturd – Ep. 706 – 12/16/2024
65.5K21