Premium Only Content
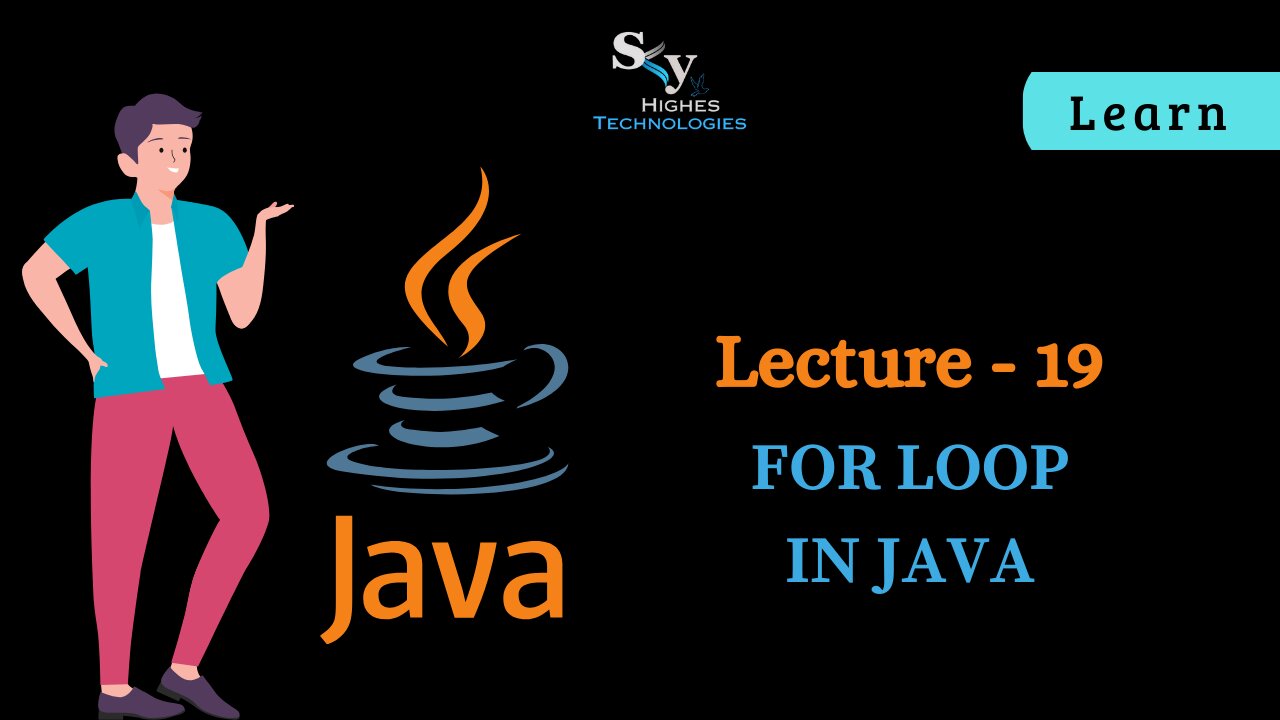
#19 For Loop in JAVA | Skyhighes | Lecture 19
For Loops in Java: The Masterful Conductors of Repetition
Imagine a skilled orchestra conductor leading musicians through a symphony's repetitive sections. The for loop is Java's conductor, effortlessly guiding your code through tasks that need to be performed a specific number of times.
Here's how it orchestrates the process:
1. Initialization:
Sets the starting point for the loop, similar to tuning instruments before a performance.
Declares and initializes a variable that will control the loop's iterations.
2. Condition Check:
Determines whether to continue the loop, like the conductor checking the sheet music for repeats.
Evaluates a boolean expression before each iteration.
If the condition is true, the loop body executes. If false, the loop terminates.
3. Code Execution:
The music happens here! The code within the loop executes, performing the desired actions.
4. Increment/Decrement:
Like the conductor guiding the musicians through each measure, this step updates the loop control variable, moving it closer to the end.
This often involves incrementing or decrementing the variable.
5. Repeat and Resolve:
The cycle of condition check, code execution, and increment/decrement continues until the condition becomes false.
Once the condition is no longer met, the loop concludes, and the program moves on to the next instructions, just as the symphony moves to its next movement.
Syntax:
Java
for (initialization; condition; increment/decrement) {
// Code to be repeated
}
Use code with caution. Learn more
Example:
Java
for (int i = 1; i <= 5; i++) {
System.out.println("Counting: " + i);
}
Use code with caution. Learn more
Key Points:
Conciseness: For loops often pack initialization, condition, and increment into a single line, making them compact and readable.
Definite Iteration: Ideal for scenarios where you know the number of repetitions beforehand.
Flexibility: Customize the initialization, condition, and increment/decrement to fit various needs.
Common Use Cases:
Iterating through arrays or collections
Printing sequences of numbers
Implementing countdowns or count-ups
Handling nested loops for complex repetition patterns
Remember: For loops are the workhorses of Java repetition. By mastering their use, you'll conduct your code through repetitive tasks with precision and elegance, composing harmonious programs that achieve their intended outcomes.
-
21:35
DeVory Darkins
3 days ago $6.89 earnedMitch McConnell TORCHED as Secretary of HHS is sworn in
21.3K99 -
1:20:04
Tim Pool
4 days agoGame of Money
53.8K10 -
2:21:11
Nerdrotic
11 hours ago $25.29 earnedDown the Rabbit Hole with Kurt Metzger | Forbidden Frontier #090
104K21 -
2:41:13
vivafrei
16 hours agoEp. 251: Bogus Social Security Payments? DOGE Lawsduit W's! Maddow Defamation! & MORE! Viva & Barnes
232K264 -
1:19:23
Josh Pate's College Football Show
9 hours ago $3.88 earnedBig Ten Program Rankings | What Is College Football? | Clemson Rage| Stadiums I Haven’t Experienced
60.7K1 -
LIVE
Vigilant News Network
15 hours agoBombshell Study Reveals Where the COVID Vaccine Deaths Are Hiding | Media Blackout
1,892 watching -
1:17:59
Sarah Westall
10 hours agoDOGE: Crime & Hysteria bringing the Critics & the Fearful - Plus new CDC/Ukraine Crime w/ Dr Fleming
56.2K3 -
45:39
Survive History
16 hours ago $9.19 earnedCould You Survive in the Shield Wall at the Battle of Hastings?
64.4K6 -
1:50:28
TheDozenPodcast
15 hours agoViolence, Abuse, Jail, Reform: Michael Maisey
101K4 -
23:01
Mrgunsngear
1 day ago $5.94 earnedWolfpack Armory AW15 MK5 AR-15 Review 🇺🇸
86.9K12