Premium Only Content
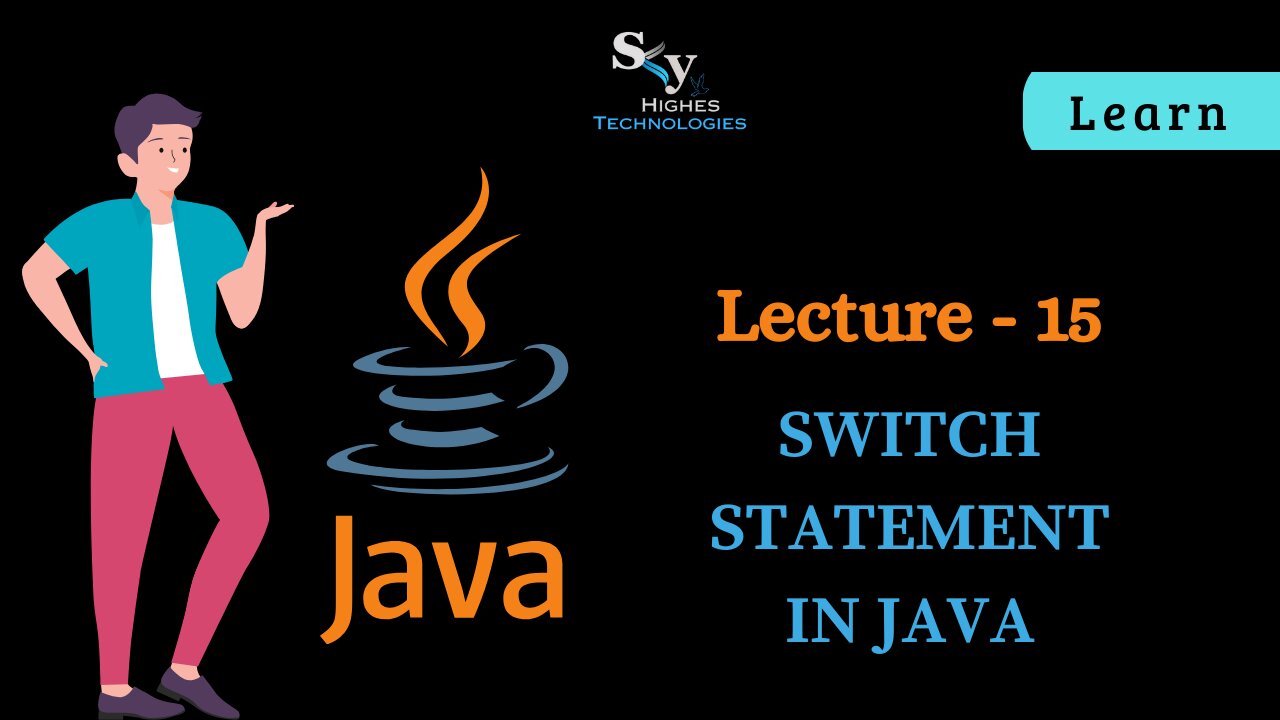
#15 Switch Statement in JAVA | Skyhighes | Lecture 15
The Switch Statement: Java's Multi-Choice Maestro
Imagine your Java code standing at a crossroads, faced with several paths to choose from. The switch statement acts as a wise guide, analyzing specific options and directing the program down the right path.
Think of it like a fancy menu:
Each option (like "spaghetti", "pizza", "salad") corresponds to a different case label.
The code evaluates the chosen dish (the input value) and executes the recipe (the code block) associated with that case.
Here's how it works:
The "Menu":
You define one or more case labels with specific values or ranges.
Think of these as the available dishes on the menu.
The "Input":
You evaluate an expression, often a variable, whose value will determine the chosen path.
Imagine this as selecting your desired dish from the menu.
The "Matching Case":
The switch statement compares the input value with each case label.
If a match is found, the code block associated with that case is executed.
The "Default Option" (Optional):
You can include a default block that runs if no case matches the input value.
Think of this as the "catch-all" option on the menu if your desired dish isn't listed.
Example:
Java
String day = "Tuesday";
switch (day) {
case "Monday":
System.out.println("It's coffee day!");
break;
case "Tuesday":
System.out.println("Taco Tuesday!");
break;
case "Wednesday":
System.out.println("Hump day...");
break;
default:
System.out.println("Just another day...");
}
Use code with caution. Learn more
Key Points:
Use the switch statement when you have a limited number of distinct options to handle.
Each case label must have a unique value or range.
Use a break statement within each case block to prevent unwanted fall-through to subsequent cases.
Consider using if-else statements for scenarios with complex conditions or many branching paths.
Remember: The switch statement is a valuable tool for handling multiple choices efficiently. Master its use, and your Java code will navigate decision-making with the elegance of a seasoned restaurateur!
Bonus Tip: For handling strings a
-
1:00:37
Bright Insight
10 days agoOlmec Heads are Evidence of Lost Ancient Advanced Civilization
66.6K207 -
13:43
Cooking with Gruel
2 days agoCreamy Saffron Risotto
47.5K10 -
18:38
DeVory Darkins
20 hours ago $12.57 earnedTrump Makes HUGE Announcement that may spark GOP Battle
35.9K56 -
2:13:05
The Nerd Realm
6 hours ago $1.77 earnedNew Years Eve! Fortnite Hunters w/ YOU! Creator Code: NERDREALM
23.8K2 -
LIVE
FusedAegisTV
22 hours agoNYE Eve! - 2025 Incoming 🎉 - 12hr Variety Stream!
442 watching -
1:18:52
Awaken With JP
9 hours agoSomehow The World DIDN’T End This Year! - LIES Ep 72
89.2K44 -
1:19:34
Michael Franzese
7 hours agoWhat 2024 Taught Us About the Future?
83.8K22 -
1:48:09
The Quartering
7 hours agoBird Flu PANIC, Sam Hyde DESTROYS Elon Musk & Patrick Bet David & Woke Witcher?
96.2K63 -
4:47
SLS - Street League Skateboarding
3 days agoLiz Akama’s 2nd Place Finish at SLS Tokyo 2024 | Best Tricks
34.1K3 -
4:06:54
LumpyPotatoX2
6 hours agoHappy New Year Rumble ! - #RumbleGaming
24K