Premium Only Content
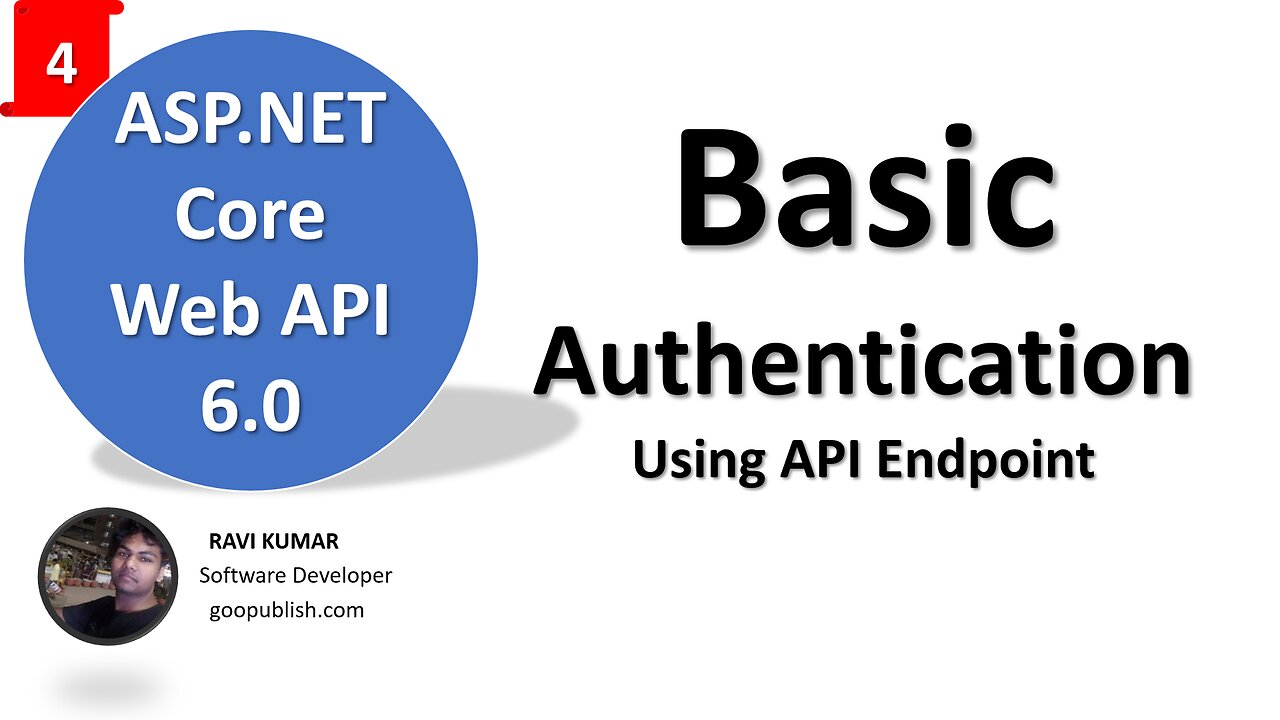
Part-4 | How to Implement Basic Authentication || Asp.Net Core Web API 6.0
Basic Authentication is a simple authentication mechanism used to secure web APIs and other online services. It involves sending a username and password in the HTTP request headers, which are base64-encoded. While it's straightforward to implement, it's not the most secure method and is typically used in combination with HTTPS to ensure the security of the transmitted credentials.
Here's how Basic Authentication works in theory using a web API:
1. **Client Request**: The client (e.g., a mobile app or a web application) makes an HTTP request to a protected resource on the web API server. The client includes an "Authorization" header in the request.
2. **Header Creation**: To create the "Authorization" header, the client combines the username and password into a single string, separated by a colon (e.g., "username:password"). This string is then base64-encoded.
3. **HTTP Request with Authorization Header**: The client includes the base64-encoded string in the "Authorization" header of the HTTP request.
4. **Server Authentication**: The server receives the HTTP request and extracts the "Authorization" header.
5. **Decoding the Credentials**: The server decodes the base64-encoded string from the "Authorization" header to obtain the username and password.
6. **Authentication**: The server compares the provided credentials with its stored user credentials (e.g., in a database). If they match, the server grants access to the protected resource. Otherwise, it returns an authentication error (e.g., HTTP 401 Unauthorized).
7. **Access Granted**: If the credentials are valid, the server allows the client to access the protected resource, and it returns the requested data or performs the requested action.
In this example, "dXNlcm5hbWU6cGFzc3dvcmQ=" is the base64-encoded string for "username:password."
Important considerations:
1. **Security**: Basic Authentication should always be used with HTTPS to encrypt the credentials during transmission. Without HTTPS, the credentials can be easily intercepted.
2. **Credential Storage**: Server-side, it's essential to securely store user credentials (usually hashed and salted) to protect them from data breaches.
3. **Token-Based Authentication**: While Basic Authentication is simple, token-based authentication methods like OAuth2 or JSON Web Tokens (JWT) are more secure and often preferred for modern web APIs.
4. **Rate Limiting**: To prevent abuse, it's a good practice to implement rate limiting and other security measures when using Basic Authentication.
5. **User Experience**: Consider user experience when using Basic Authentication, as it may require users to enter their credentials frequently, unlike token-based methods that offer more persistent sessions.
-
LIVE
Right Side Broadcasting Network
7 days agoLIVE: TPUSA's America Fest Conference: Day One - 12/19/24
7,475 watching -
Roseanne Barr
4 hours ago $8.51 earned"Ain't Nobody Good" with Jesse Lee Peterson | The Roseanne Barr Podcast #79
29.5K14 -
The StoneZONE with Roger Stone
1 hour agoTrump Should Sue Billionaire Governor JB Pritzker for Calling Him a Rapist | The StoneZONE
3.72K1 -
LIVE
LittleSaltyBear
2 hours ago $0.88 earnedNecromancing Path of Exile 2 4K
288 watching -
LIVE
Akademiks
2 hours agoJay Z War against Diddy Accuser Lawyer GOES CRAZY! Lil Baby Speaks OUT! Cardi v Offset? Bhad Bhabie?
4,168 watching -
LIVE
Josh Pate's College Football Show
3 hours ago $0.09 earnedCFP Changes Coming | Transfer Portal Intel | Games Of The Year | Head Coaches Set To Elevate
56 watching -
1:21:17
Donald Trump Jr.
6 hours agoWhat are These Mystery Drones? Plus Inside the Swamp’s CR. Interview with Lue Elizondo | TRIGGERED Ep.200
96K95 -
37:54
Kimberly Guilfoyle
6 hours agoAmerica is Healing, Plus Fani Willis Disqualified, Live with Shemane Nugent & Mike Davis | Ep. 182
72.8K39 -
7:38
Game On!
4 hours ago $0.48 earnedThe picks you need for Thursday Night Football!
11.6K -
55:30
LFA TV
1 day agoBible Prophecy Was Center Stage in 2024 | Trumpet Daily 12.19.24 7PM EST
23.6K1