Premium Only Content
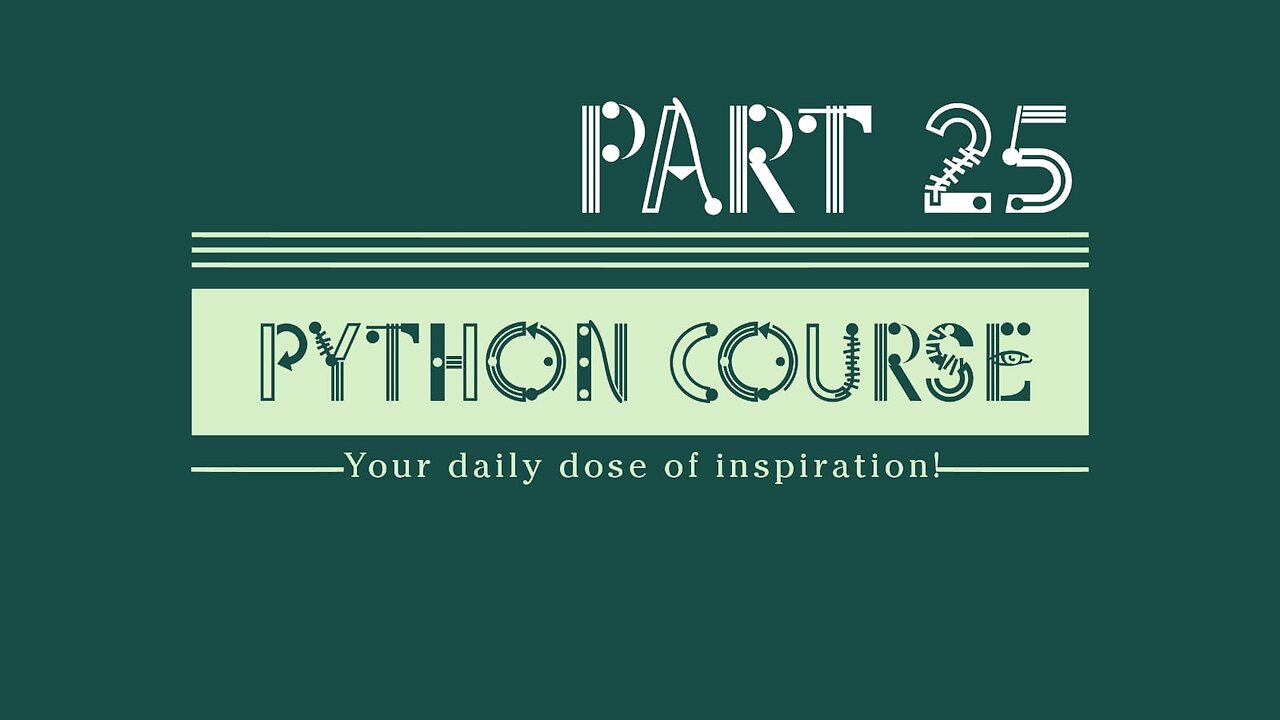
Functions and User Input |Section 3|Celestial Warrior
The next lecture will be an exercise and there will be more exercises as you progress through the course.
The exercises are difficult and sometimes tricky and I don't expect students to solve them all. Most students actually fail to solve the exercisesunless they are already familiar with Python.
The whole point of exercises is to force you to think about how to solve a problem independently. The next lecture following each exercise contains the solution where you can compare your approach.
Create a functionthat converts Celsius degrees toFahrenheit. The formula to convert Celsius to Fahrenheit isF= C 9/5 + 32.
In the previous exercises you used the interactive Udemy interface to write your code.For this exercise, please use your computertools (Python, editor, terminal,etc.). This will help you get used with Python in a real environment.
Once you are done check your solution against my solution which islocatedin the next lecture. Don't worry if your solution is not exactly the same as mine. As far as the solutions generate the same output, your solution is most likely correct.
def cel_to_fahr(c):
f = c * 9/5 + 32
return f
And here is an example of calling the function:
print(cel_to_fahr(10))
That would return 50.0 which means 10 degreeCelsius is equal to 50 degreeFahrenheit.
Create a function that takes any string as argument and returns the length of that string.
def string_length(mystring):
return len(mystring)
And here's an example of calling the function:
print(string_length("Hello"))
That would return 5.
-
3:58
Blackstone Griddles
23 hours agoLeftover Turkey with White Country Gravy
27.8K3 -
42:19
Lights, Camera, Barstool
9 hours agoDoes 'Glicked' Meet The Hype? 'Gladiator II' And 'Wicked' Reviews
27.6K2 -
1:28:03
MTNTOUGH Fitness Lab
2 hours agoRandy Newberg's Shot of a Lifetime: The Intense 5-Second Window for a Trophy Ram | MTNT POD #91
13.5K -
1:02:44
World Nomac
10 hours agoThe side of Las Vegas they don't want you to know about
10.7K -
1:56:32
TheSaf3Hav3n
3 hours ago| CALL OF DUTY: BLACK OPS 6 - NUKETOWN | GET IN HERE!! | #RumbleTakeOver |
16.8K -
LIVE
MissesMaam
6 hours agoMY FAVORITE ARTIST IS FINALLY IN FORTNITE 💚✨
86 watching -
2:02:34
The Quartering
5 hours agoTrump Tariffs Immediately Work, Thanksgiving Cost Insanity, Hollywood Actor In Psych Ward From Trump
82.2K32 -
36:54
Stephen Gardner
4 hours ago🔥I can't believe what Happened To Trump's insider pick!
22.2K48 -
27:59
LumpyPotatoX2
20 hours agoRumble Gaming Talk W/Chris Pavlovski & SilverFox - #RumbleGaming
40.1K8 -
1:50:13
vivafrei
6 hours agoInterview with Rumble CEO Chris Pavlovski - Rumble & Bitcoin? Shocking Stats From Florida & MORE!
91.3K16