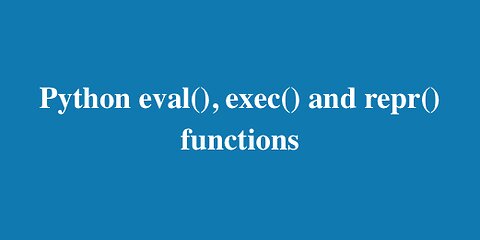
-
33. Python: eval(),exec(),repr() function
Python Beyond Basics for Machine Learning, Data Science, AI* The eval() function evaluates the specified expression, if the expression is a legal Python statement, it will be executed. * The exec() function executes the specified Python code. The exec() function accepts large blocks of code, unlike the eval() function which only accepts a single expression. * The repr() function returns a string containing a printable representation of an object.77 views 1 comment -
31. Python: isinstance, Use of format, Timeit, round, Slice and abs
Python Beyond Basics for Machine Learning, Data Science, AI1. isinstance() function returns True if the specified object is of the specified type, otherwise False. If the type parameter is a tuple, this function will return True if the object is one of the types in the tuple. 2. format() method formats the specified value(s) and insert them inside the string's placeholder. The placeholder is defined using curly brackets: {}. Read more about the placeholders in the Placeholder section below. format() method returns the formatted string. 3. timeit() method measure execution time of small code snippets. This module provides a simple way to time small bits of Python code. 4. round() function returns a floating point number that is a rounded version of the specified number, with the specified number of decimals. The default number of decimals is 0, meaning that the function will return the nearest integer. 5. slice() function returns a slice object. A slice object is used to specify how to slice a sequence. You can specify where to start the slicing, and where to end. You can also specify the step, which allows you to e.g. slice only every other item. 6. abs() function returns the absolute value of the specified number.104 views 1 comment -
30. Python Queue
Python Beyond Basics for Machine Learning, Data Science, AIIt is an in-built module for implementing a queue in Python. You can use different functions available in the module to perform operations on a queue. Below is an example of implementing a queue with the help of a queue, along with the use of different functions.225 views 1 comment -
29. Python: Lambda, Map, Filter and Reduce Function.
Python Beyond Basics for Machine Learning, Data Science, AITo work with map(), the lambda should have one parameter in, representing one element from the source list. Choose a suitable name for the parameter, like n for a list of numbers, s for a list of strings. The result of map() is an "iterable" map object which mostly works like a list, but it does not print.182 views -
24. Python: User-defined functions and Inbuilt functions
Python Beyond Basics for Machine Learning, Data Science, AIIf you define the function yourself, it is a user-defined function. On the other hand, the Python function that come along with Python are known as in-built functions. All the functions apart from in-built functions and library functions come under the category of user-defined functions.108 views -
25. Python: iterator
Python Beyond Basics for Machine Learning, Data Science, AIAn iterator is an object that contains a countable number of values. An iterator is an object that can be iterated upon, meaning that you can traverse through all the values. Technically, in Python, an iterator is an object which implements the iterator protocol, which consist of the methods __iter__() and __next__().126 views -
28. Python Logging Module
Python Beyond Basics for Machine Learning, Data Science, AIPython logging is a module that allows you to track events that occur while your program is running. You can use logging to record information about errors, warnings, and other events that occur during program execution. And logging is a useful tool for debugging, troubleshooting, and monitoring your program.299 views -
27. Python: Global and local Variables in Functions.
Python Beyond Basics for Machine Learning, Data Science, AILocal variables exist only during the function's executions, while global variables remain in memory for the duration of the program. Local variables can be accessed only within the function or block where it is defined, whereas global variables are accessed throughout the entire program.112 views 1 comment -
26. Python: Generator and decorators.
Python Beyond Basics for Machine Learning, Data Science, AIDecorators and generators are powerful concepts in Python that enhance code reusability, readability, and performance. Decorators let you add functionality before and/or after function execution dynamically, while generators enable the generation of sequence values on the fly instead of loading them all at once.200 views -
23. Python CSV file Operations
Python Beyond Basics for Machine Learning, Data Science, AIReading from a CSV file is done using the reader object. The CSV file is opened as a text file with Python's built-in open() function, which returns a file object. This is then passed to the reader , which does the heavy lifting.96 views